Developer Guide
- Acknowledgements
- Setting up, getting started
- Design
- Implementation
- Documentation, logging, testing, configuration, dev-ops
- Appendix: Requirements
-
Appendix: Instructions for manual testing
- Launch and shutdown
- Student Test Cases
- Adding a student
- Finding a student (by course)
- Finding a student (by name)
- Editing a student
- Deleting a student
- Lesson Test Cases
- Adding a lesson:
addlesson
- Adding a student to a lesson:
addtolesson
- Removing a student from a lesson:
removefromlesson
- Marking attendance in a lesson:
marka
- Marking participation in a lesson:
markp
- Removing a lesson:
deletelesson
- Consultation Test Cases
- Adding a consultation:
addconsult
- Listing all consultations:
listconsults
- Adding students to a consultation:
addtoconsult
- Removing students from a consultation:
removefromconsult
- Deleting consultations:
deleteconsult
- Saving data
- Exporting data
- Importing data
Acknowledgements
- {list here sources of all reused/adapted ideas, code, documentation, and third-party libraries – include links to the original source as well}
Setting up, getting started
Refer to the guide Setting up and getting started.
Design

.puml
files used to create diagrams in this document docs/diagrams
folder. Refer to the PlantUML Tutorial at se-edu/guides to learn how to create and edit diagrams.
Architecture
The Architecture Diagram given above explains the high-level design of the App.
Given below is a quick overview of main components and how they interact with each other.
Main components of the architecture
Main
(consisting of classes Main
and MainApp
) is in charge of the app launch and shut down.
- At app launch, it initializes the other components in the correct sequence, and connects them up with each other.
- At shut down, it shuts down the other components and invokes cleanup methods where necessary.
The bulk of the app’s work is done by the following four components:
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
Commons
represents a collection of classes used by multiple other components.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
Each of the four main components (also shown in the diagram above),
- defines its API in an
interface
with the same name as the Component. - implements its functionality using a concrete
{Component Name}Manager
class (which follows the corresponding APIinterface
mentioned in the previous point).
For example, the Logic
component defines its API in the Logic.java
interface and implements its functionality using the LogicManager.java
class which follows the Logic
interface. Other components interact with a given component through its interface rather than the concrete class (reason: to prevent outside component’s being coupled to the implementation of a component), as illustrated in the (partial) class diagram below.
The sections below give more details of each component.
UI component
The API of this component is specified in Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, StudentListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class which captures the commonalities between classes that represent parts of the visible GUI.
The UI
component uses the JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- executes user commands using the
Logic
component. - listens for changes to
Model
data so that the UI can be updated with the modified data. - keeps a reference to the
Logic
component, because theUI
relies on theLogic
to execute commands. - depends on some classes in the
Model
component, as it displaysStudent
,Consultation
&Lesson
objects residing in theModel
.
Logic component
API : Logic.java
Here is a fuller diagram of how the Logic
component might interact with adjacent classes:
The sequence diagram below illustrates the interactions within the Logic
component, taking execute("delete 1")
API call as an example.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline continues till the end of diagram.
How the Logic
component works:
- When
Logic
is called upon to execute a command, it is passed to anAddressBookParser
object which in turn creates a parser that matches the command (e.g.,DeleteCommandParser
) and uses it to parse the command. - This results in a
Command
object (more precisely, an object of one of its subclasses e.g.,DeleteCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to delete a student).
Note that although this is shown as a single step in the diagram above (for simplicity), in the code it can take several interactions (between the command object and theModel
) to achieve. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Here are the other classes in Logic
(omitted from the class diagram above) that are used for parsing a user command:
How the parsing works:
- When called upon to parse a user command, the
AddressBookParser
class creates anXYZCommandParser
(XYZ
is a placeholder for the specific command name e.g.,AddCommandParser
) which uses the other classes shown above to parse the user command and create aXYZCommand
object (e.g.,AddCommand
) which theAddressBookParser
returns back as aCommand
object. - All
XYZCommandParser
classes (e.g.,AddCommandParser
,DeleteCommandParser
, …) inherit from theParser
interface so that they can be treated similarly where possible e.g, during testing.
Model component
API : Model.java
The Model
component,
- stores the address book data i.e., all
Student
objects (which are contained in aUniqueStudentList
object). - stores the currently ‘selected’
Student
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Student>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores the address book data i.e., all
Consultation
objects (which are contained in aUniqueConsultList
object). - stores the currently ‘selected’
Consultation
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Consultation>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores the address book data i.e., all
Lesson
objects (which are contained in aUniqueLessonList
object). - stores the currently ‘selected’
Lesson
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Lesson>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores a
UserPref
object that represents the user’s preferences. This is exposed to the outside as aReadOnlyUserPref
objects. - does not depend on any of the other three components (as the
Model
represents data entities of the domain, they should make sense on their own without depending on other components)

Course
list in the AddressBook
, which Student
references. This allows AddressBook
to only require one Course
object per unique Course, instead of each Student
needing their own Course
objects.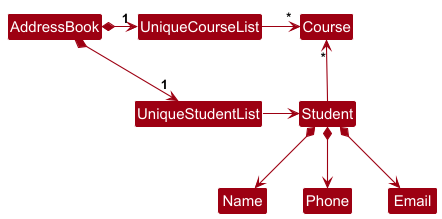
Storage component
API : Storage.java
The Storage
component,
- can save both address book data and user preference data in JSON format, and read them back into corresponding objects.
- inherits from both
AddressBookStorage
andUserPrefStorage
, which means it can be treated as either one (if only the functionality of only one is needed). - depends on some classes in the
Model
component (because theStorage
component’s job is to save/retrieve objects that belong to theModel
)
Common classes
Classes used by multiple components are in the seedu.address.commons
package.
Implementation
This section describes some noteworthy details on how certain features are implemented.
Consultation Management
The consultation management feature enables TAs to schedule and manage consultation sessions with students. This section describes the implementation details of the consultation system.
Architecture
The consultation feature comprises these key components:
-
Consultation
: Core class representing a consultation session -
Date
: Represents and validates consultation dates -
Time
: Represents and validates consultation times -
AddConsultCommand
: Handles adding new consultations -
AddConsultCommandParser
: Parses user input for consultation commands
The class diagram below shows the structure of the consultation feature:
Implementation
The consultation management system is implemented through several key mechanisms:
1. Date and Time Validation
The system enforces strict validation for consultation scheduling:
- Dates must be in
YYYY-MM-DD
format and represent valid calendar dates - Times must be in 24-hour
HH:mm
format - Both use Java’s built-in
LocalDate
andLocalTime
for validation
Example:
Date date = new Date("2024-10-20"); // Valid
Time time = new Time("14:00"); // Valid
Date invalidDate = new Date("2024-13-45"); // Throws IllegalArgumentException
2. Consultation Management
The Consultation
class manages:
- Immutable date and time properties
- Thread-safe student list management
- Equality based on date, time, and enrolled students
Core operations:
// Creating a consultation
Consultation consult = new Consultation(date, time, students);
// Adding/removing students
consult.addStudent(student);
consult.removeStudent(student);
// Getting immutable student list
List<Student> students = consult.getStudents(); // Returns unmodifiable list
The sequence diagram below shows how the addStudent(student)
method is performed in the Consultation
class.
The command first checks if the student is already in the consultation. If the student was already in the consultation, and exception is thrown and the student is not added.
However, if the student was not previously in the consultation, the student is now added to the list of students in the consultation.
3. Command Processing
The system supports these consultation management commands:
-
addconsult
: Creates new consultation sessions -
addtoconsult
: Adds students to existing consultations -
deleteconsult
: Removes consultation sessions -
removefromconsult
: Removes students from consultations
Command examples:
addconsult d/2024-10-20 t/14:00
addtoconsult 1 n/John Doe i/3
deleteconsult 1
removefromconsult 1 n/John Doe
The sequence diagram below shows how the command addtoconsult 1 n/John Doe i/3
is executed.
The commands for Consultations are executed using the Logic
component:
- When
Logic
is called upon to execute a command, it is passed to anAddressBookParser
object which in turn creates a parser that matches the command (e.g.,AddToConsultCommandParser
) and uses it to parse the command. - This results in a
Command
object (in this case, theAddToConsultCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to add a student to the consultation).
Note that although this is shown as a single step in the diagram above (for simplicity), in the code it can take several interactions (between the command object and theModel
) to achieve. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Aspect 1: Date and Time Representation
-
Alternative 1 (current choice): Separate
Date
andTime
classes- Pros: Clear separation of concerns, focused validation
- Cons: Two objects to manage instead of one
-
Alternative 2: Combined
DateTime
class- Pros: Unified handling of temporal data
- Cons: More complex validation, reduced modularity
Aspect 2: Student List Management
-
Alternative 1 (current choice): Immutable view with mutable internal list
- Pros: Thread-safe external access, flexible internal updates
- Cons: Complex implementation
-
Alternative 2: Fully immutable list
- Pros: Simpler thread-safety
- Cons: Higher memory usage for modifications
Lesson Management
The lesson management feature enables TAs to schedule and manage lessons with students. This section describes the implementation details of the lesson system.
Architecture
The lesson feature comprises these key components:
-
Lesson
: Core class representing a lessons -
Date
: Represents and validates lesson dates -
Time
: Represents and validates lesson times -
StudentLessonInfo
: Represents student info used for lessons. This includes the student’s attendance and participation score for a lesson. -
AddLessonCommand
: Handles adding new lessons -
AddLessonCommandParser
: Parses user input forAddLessonCommand
The class diagram below shows the structure of the lesson feature:
Implementation
The lesson management system is implemented through several key mechanisms:
1. Date and Time Validation
The system enforces strict validation for lesson scheduling:
- Dates must be in
YYYY-MM-DD
format and represent valid calendar dates - Times must be in 24-hour
HH:mm
format - Both use Java’s built-in
LocalDate
andLocalTime
for validation
Example:
Date date = new Date("2024-10-20"); // Valid
Time time = new Time("14:00"); // Valid
Date invalidDate = new Date("2024-13-45"); // Throws IllegalArgumentException
2. Lesson Management
The Lesson
class manages:
- Immutable date and time properties
- Thread-safe student information list management
- Equality based on date, time, and student information
Core operations:
// Creating a lesson
Lesson lesson = new Lesson(date, time, studentLessonInfoList);
// Adding/removing students
lesson.addStudent(student);
lesson.removeStudent(student);
// Setting attendance
lesson.setAttendance(student, attendance);
// Setting pariticipation score
lesson.setParticipation(student, participationScore);
// Getting immutable student info list, returns unmodifiable list
List<StudentLessonInfo> studentInfoList = lesson.getStudentLessonInfoList();
3. Command Processing
The system supports these lesson management commands:
-
addlesson
: Creates new lesson -
addtolesson
: Adds students to existing lesson -
deletelesson
: Removes lesson -
listlessons
: Lists all lessons -
removefromlesson
: Removes students from lesson -
marka
: Marks the attendance of students in a lesson (can mark as present or absent) -
markp
: Sets the participation score of students in a lesson
Command examples:
addlesson d/2024-10-20 t/14:00
addtolesson 1 n/John Doe i/3
deletelesson 1
removefromlesson 1 n/John Doe
markp 1 n/John Doe pt/25
marka 1 n/John Doe n/Jane Doe a/y
The sequence diagram below shows how the command addtolesson 1 n/John Doe i/3
is executed.
The commands for Lessons are executed using the Logic
component, making it similar to the sequence diagram in the consultation section above.
- When
Logic
is called upon to execute a command, it is passed to anAddressBookParser
object which in turn creates a parser that matches the command (e.g.,AddToLessonCommandParser
) and uses it to parse the command. - This results in a
Command
object (in this case, theAddToLessonCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to add a student to the lesson).
Note that although this is shown as a single step in the diagram above (for simplicity), in the code it can take several interactions (between the command object and theModel
) to achieve. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
The next sequence diagram shows how the MarkLessonAttendanceCommand
is executed to update the attendance of students in a lesson.
The flow of the program when MarkLessonAttendanceCommand.execute
is called is as follows:
- When the execute method is called, the command first gets the
targetLesson
from the model. - The command then creates a new
Lesson
object (callednewLesson
) from thetargetLesson
. This creates a copy of the currenttargetLesson
. - Now, for each student, the command sets the student’s attendance using the
setAttendance
method in theLesson
class. - Finally, the command replaces the
targetLesson
in the model with thenewLesson
.
Aspect 1: Date and Time Representation
-
Alternative 1 (current choice): Separate
Date
andTime
classes- Pros: Clear separation of concerns, focused validation
- Cons: Two objects to manage instead of one
-
Alternative 2: Combined
DateTime
class- Pros: Unified handling of temporal data
- Cons: More complex validation, reduced modularity
Aspect 2: StudentInfo List Management
-
Alternative 1 (current choice): Immutable view with mutable internal list
- Pros: Thread-safe external access, flexible internal updates
- Cons: Complex implementation
-
Alternative 2: Fully immutable list
- Pros: Simpler thread-safety
- Cons: Higher memory usage for modifications
Data Import / Export Feature
The import/export feature allows TAs to archive and transfer their data in CSV format. This functionality is implemented for both students and consultations.
Implementation
The feature is implemented through four main command classes:
-
ExportCommand
: Exports student data to CSV -
ExportConsultCommand
: Exports consultation data to CSV -
ImportCommand
: Imports student data from CSV -
ImportConsultCommand
: Imports consultation data from CSV
Export File Handling:
- Files are always saved in the
data
directory first as the primary storage location - The command then attempts to copy the file to the user’s home directory as a backup
- If the home directory copy fails, the command still succeeds with only the data directory file
- The -f flag allows overwriting of existing files in both locations
- Filenames are restricted to alphanumeric characters to prevent path traversal attacks and ensure cross-platform compatibility
Import File Resolution:
The system searches for import files in this priority order to balance security and convenience:
- Data directory (./data/) - primary application storage
- Current directory - for convenience during testing/development
- Home directory paths (with ~) - for user convenience
- Absolute paths - for flexibility in file locations
Each export command follows this workflow:
- Validates filename input (must be alphanumeric))
- Creates
data
directory if needed - Writes data to CSV in
data
directory - Copies file to home directory if possible
- Handles file overwrite with force flag (-f)
- Returns success with one or both file paths
Each import command follows this workflow:
- Resolves file path using priority order:
- Data directory (./data/)
- Current directory
- Home directory paths (with ~)
- Absolute paths
- Validates file exists and is readable
- Parses CSV header
- Process entries line by line
- Logs invalid entries to
error.csv
Example sequences:
Student CSV format:
Name,Phone,Email,Courses
John Doe,12345678,john@example.com,CS2103T;CS2101
Consultation CSV format:
Date,Time,Students
2024-10-20,14:00,John Doe;Jane Doe
Design Considerations
Aspect: File Format
-
Alternative 1 (current choice): CSV format
- Pros: Widely compatible, human-readable, easy to edit
- Cons: Limited structure, requires careful escaping
-
Alternative 2: JSON format
- Pros: Maintains data structure, less escaping needed
- Cons: Less human-readable, harder to edit manually
Aspect: Error Handling
-
Alternative 1 (current choice): Log errors to separate file
- Pros: Clear error reporting, allows partial imports
- Cons: Requires managing separate error log file
-
Alternative 2: Fail entire import on any error
- Pros: Ensures data consistency
- Cons: Less flexible, requires perfect input
Aspect: Export File Location
-
Alternative 1 (current choice): Dual location with strict filenames
- Pros: Convenient access, automatic backup
- Cons: More complex validation, potential sync issues
-
Alternative 2: Single location with flexible paths
- Pros: Simpler implementation, more user control
- Cons: Manual backup required
[Proposed] Undo/redo feature
Proposed Implementation
The proposed undo/redo mechanism is facilitated by VersionedAddressBook
. It extends AddressBook
with an undo/redo history, stored internally as an addressBookStateList
and currentStatePointer
. Additionally, it implements the following operations:
-
VersionedAddressBook#commit()
— Saves the current address book state in its history. -
VersionedAddressBook#undo()
— Restores the previous address book state from its history. -
VersionedAddressBook#redo()
— Restores a previously undone address book state from its history.
These operations are exposed in the Model
interface as Model#commitAddressBook()
, Model#undoAddressBook()
and Model#redoAddressBook()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedAddressBook
will be initialized with the initial address book state, and the currentStatePointer
pointing to that single address book state.
Step 2. The user executes delete 5
command to delete the 5th student in the address book. The delete
command calls Model#commitAddressBook()
, causing the modified state of the address book after the delete 5
command executes to be saved in the addressBookStateList
, and the currentStatePointer
is shifted to the newly inserted address book state.
Step 3. The user executes add n/David …
to add a new student. The add
command also calls Model#commitAddressBook()
, causing another modified address book state to be saved into the addressBookStateList
.

Model#commitAddressBook()
, so the address book state will not be saved into the addressBookStateList
.
Step 4. The user now decides that adding the student was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoAddressBook()
, which will shift the currentStatePointer
once to the left, pointing it to the previous address book state, and restores the address book to that state.

currentStatePointer
is at index 0, pointing to the initial AddressBook state, then there are no previous AddressBook states to restore. The undo
command uses Model#canUndoAddressBook()
to check if this is the case. If so, it will return an error to the user rather
than attempting to perform the undo.
The following sequence diagram shows how an undo operation goes through the Logic
component:

UndoCommand
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
Similarly, how an undo operation goes through the Model
component is shown below:
The redo
command does the opposite — it calls Model#redoAddressBook()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the address book to that state.

currentStatePointer
is at index addressBookStateList.size() - 1
, pointing to the latest address book state, then there are no undone AddressBook states to restore. The redo
command uses Model#canRedoAddressBook()
to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
Step 5. The user then decides to execute the command liststudents
. Commands that do not modify the address book, such as liststudents
, will usually not call Model#commitAddressBook()
, Model#undoAddressBook()
or Model#redoAddressBook()
. Thus, the addressBookStateList
remains unchanged.
Step 6. The user executes clear
, which calls Model#commitAddressBook()
. Since the currentStatePointer
is not pointing at the end of the addressBookStateList
, all address book states after the currentStatePointer
will be purged. Reason: It no longer makes sense to redo the add n/David …
command. This is the behavior that most modern desktop applications follow.
The following activity diagram summarizes what happens when a user executes a new command:
Design considerations:
Aspect: How undo & redo executes:
-
Alternative 1 (current choice): Saves the entire address book.
- Pros: Easy to implement.
- Cons: May have performance issues in terms of memory usage.
-
Alternative 2: Individual command knows how to undo/redo by
itself.
- Pros: Will use less memory (e.g. for
delete
, just save the student being deleted). - Cons: We must ensure that the implementation of each individual command are correct.
- Pros: Will use less memory (e.g. for
Documentation, logging, testing, configuration, dev-ops
Appendix: Requirements
Product scope
Target user profile:
Teaching Assistants (TAs) in academic institutions such as universities, colleges, and online learning platforms.
- Role: TAs supporting professors and lecturers in managing course-related tasks.
- Experience Level: New and experienced TAs handling multiple responsibilities.
- Needs: Efficient management of student information and tasks.
- Has a need to manage a significant number of contacts
- Prefer desktop apps over other types
- Can type fast
- Prefers typing to mouse interactions
- Is reasonably comfortable using CLI apps
Value proposition: TAHub simplifies the role of Teaching Assistants by providing a centralized hub to organize student information, and efficiently manage course-related tasks. This platform empowers TAs to focus more on enhancing student learning and less on administrative chaos.
User stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can … |
---|---|---|---|
* * * |
teaching assistant | add students to my course roster | keep track of all students under my supervision |
* * * |
teaching assistant | update student information | keep student records up-to-date and accurate |
* * * |
teaching assistant | search for a student by their name | quickly find a specific student when needed |
* * * |
teaching assistant | search for students enrolled in a course | quickly find all students enrolled in a certain course |
* * * |
teaching assistant | filter students by homework submission status | quickly get to grading and providing feedback |
* * * |
teaching assistant | mark students’ attendance in my tutorial | leverage my fast typing to quickly take attendance |
* * * |
teaching assistant | assign participation marks to each student | keep track of student participation easily to assign a grade later |
* * * |
teaching assistant | export student data as a CSV | easily share information with professors or use in other applications |
* * * |
teaching assistant | schedule consultation sessions | set aside dedicated time slots to meet with students |
* * * |
teaching assistant | add students to consultation slots | keep track of which students are attending each consultation |
* * * |
teaching assistant | view all my upcoming consultations | prepare for and manage my consultation schedule |
* * |
teaching assistant | assign tasks and deadlines to students | track their responsibilities and ensure they stay on schedule |
* * |
teaching assistant | set reminders for important tasks or deadlines | stay notified of upcoming responsibilities and avoid missing them |
* * |
teaching assistant | view a calendar showing all upcoming student deadlines and my TA duties | manage my time effectively and avoid scheduling conflicts |
* * |
teaching assistant | filter students by academic performance | prioritise communication with students in need of help |
* * |
teaching assistant | manually tag students in need of help | remember to give these students additional support to help them catch up |
* * |
teaching assistant | filter students by their attendance | reach out to them if they have not attended for long periods of time |
* * |
teaching assistant | mark students who will be absent with a valid reason | keep track of special cases when taking attendance |
* * |
teaching assistant | search for the availability of students during a certain time | find the preferred timing to host a consultation session |
* * |
teaching assistant | view a list of students that match my search query without typing in full | handle mass search queries for convenience |
* * |
teaching assistant | merge duplicate student entries | maintain a clean and accurate database |
* * |
teaching assistant | backup my student database to a local file | ensure data safety and practice file management |
* * |
teaching assistant | use a command to import student data from a CSV file | quickly populate my database at the start of a semester |
* * |
teaching assistant | get alerts for consultation timing conflicts | avoid double booking consultation slots |
* * |
teaching assistant | see the history of consultations with each student | track how often I meet with specific students |
* * |
teaching assistant | add notes to consultation sessions | record what was discussed and any follow-up actions needed |
* |
teaching assistant | add notes to a student’s profile | keep track of special considerations for each student |
* |
teaching assistant | assign a profile picture to each student | have a visual aid to recognise who is who in my tutorial |
* |
teaching assistant | toggle between light and dark mode | select my preferred viewing mode |
* |
teaching assistant | redesign the TAHub GUI layout | select my preferred visual layout |
* |
teaching assistant | press up and down to navigate command history | quickly reuse recently used commands |
* |
teaching assistant | generate a statistical summary of class performance | quickly assess overall class trends in scores/attendance etc. |
* |
teaching assistant | export my consultation schedule to my calendar | integrate consultation timings with my other appointments |
* |
teaching assistant | set recurring consultation slots | establish regular consultation hours without manual scheduling |
{More to be added}
Use cases
(For all use cases below, the System is the TAHub
and the Actor is the user
, unless specified otherwise)
Use case: UC1 - Add a student
MSS:
- User requests to add a student by providing the necessary details (name, contact, courses, email).
- TAHub validates the inputs.
- TAHub adds the student with the provided details.
- TAHub displays the updated student list.
Use case ends.
Extensions:
- 2a. One or more input parameters are missing or invalid.
- 2a1. TAHub shows an error message indicating the missing or invalid field(s).
Use case ends.
- 2a1. TAHub shows an error message indicating the missing or invalid field(s).
- 2b. Duplicate Student Exists (Student name matches an existing student).
- 2b1. TAHub shows a duplicate error message.
Use case ends.
- 2b1. TAHub shows a duplicate error message.
Use case: UC2 - Find students by Course
MSS:
- User requests to find students enrolled in a particular course.
- TAHub shows a list of students enrolled in that particular course.
Use case ends.
Extensions:
- 1a. There are no students enrolled in the given course.
- 1a1. TAHub shows a message indicating there are no students found.
Use case ends.
- 1a1. TAHub shows a message indicating there are no students found.
- 1b. There are multiple courses containing the given string as a prefix.
- 1b1. TAHub displays a list of all students enrolled in those courses.
Use case ends.
- 1b1. TAHub displays a list of all students enrolled in those courses.
Use case: UC3 - Find student by name
MSS:
- User requests to find a student by name.
- TAHub displays a list of students whose names contain the input string as a prefix.
Use case ends.
Extensions:
- 1a. The list is empty.
- 1a1. TAHub displays a message that there were no students found.
Use case ends.
- 1a1. TAHub displays a message that there were no students found.
Use case: UC4 - Edit Student Information
Precondition: The Student exists in TAHub.
MSS:
- User requests to edit a student’s information by providing the index and necessary details (name, contact, courses, email).
- TAHub validates the inputs.
- TAHub updates the student with the provided details.
- TAHub displays the updated student’s information.
Use case ends.
Extensions:
- 2a. One or more input parameters are missing or invalid.
- 2a1. TAHub shows an error message indicating the missing or invalid field(s).
Use case ends.
- 2a1. TAHub shows an error message indicating the missing or invalid field(s).
Use case: UC5 - Delete Student
Precondition: The Student exists in TAHub.
MSS:
- User requests to delete a specific student in the list by index.
- TAHub verifies the given index.
- TAHub deletes the student at the index in the list.
Use case ends.
Extensions:
- 2a. The given index is invalid.
- 2a1. TAHub shows an error message stating that the index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the index is invalid.
Use case: UC6 - Export Student Data
MSS:
- User requests to export student data with desired filename.
- TAHub validates filename.
- TAHub creates CSV file in data directory.
- TAHub copies file to home directory.
- TAHub shows number of students exported.
Extensions:
- 2a. Invalid filename
- 2a1. TAHub shows error message about invalid characters.
Use case ends.
- 2a1. TAHub shows error message about invalid characters.
- 3a. File already exists.
- 3a1. TAHub shows error message suggesting force flag.
Use case ends.
- 3a1. TAHub shows error message suggesting force flag.
- 3b. Directory creation fails.
- 3b1. TAHub shows error message about directory creation.
Use case ends.
- 3b1. TAHub shows error message about directory creation.
- 4a. Write operation fails.
- 4a1. TAHub shows error message about write failure.
Use case ends.
- 4a1. TAHub shows error message about write failure.
Use case: UC7 - Import Student Data
MSS:
- User requests to import student data with CSV filename.
- TAHub validates file exists and is readable.
- TAHub reads CSV header and validates format.
- TAHub processes each row and adds valid students.
- TAHub shows number of students imported and any errors.
Use case ends.
Extensions:
- 2a. File not found.
- 2a1. TAHub shows error message about missing file.
Use case ends.
- 2a1. TAHub shows error message about missing file.
- 3a. Invalid header format.
- 3a1. TAHub shows error message about expected format.
Use case ends.
- 3a1. TAHub shows error message about expected format.
- 4a. Invalid data in rows.
- 4a1. TAHub logs invalid entries to error.csv.
- 4a2. TAHub continues processing remaining rows.
- 4a3. Success message includes count of errors.
Use case ends.
Use case: UC8 - Refresh Student List
Guarantees:
- Overall Student List will be displayed.
MSS:
- User requests to refresh student list.
- TAHub refreshes and displays the student list.
Use case ends.
Use case: UC9 - Create a Consultation
MSS:
- User requests to add a consultation by providing the necessary details (date, time).
- TAHub validates the inputs.
- TAHub adds the consultation with the provided details.
- TAHub displays the updated consultation list.
Use case ends.
Extensions:
- 2a. One or more input parameters are missing or invalid.
- 2a1. TAHub shows an error message indicating the missing or invalid fields.
Use case ends.
- 2a1. TAHub shows an error message indicating the missing or invalid fields.
- 2b. Duplicate consultation Exists (Consultation date & time matches an existing consultation)
- 2b1. TAHub shows a duplicate error message.
Use case ends.
- 2b1. TAHub shows a duplicate error message.
Use case: UC10 - Add Student to Consultation
Precondition: The Consultation exists in TAHub.
MSS:
- User requests to add a specific student to the consultation by providing the necessary details (Consultation Index, Student Index, Student Name)
- TAHub validates the inputs.
- TAHub adds the student to the consultation.
- TAHub displays the updated consultation list.
Use case ends.
Extensions:
- 2a. Invalid Consultation Index.
- 2a1. TAHub shows an error message stating that the Consultation Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Consultation Index is invalid.
- 2b. Invalid Student Index.
- 2b1. TAHub shows an error message stating that the Student Index is invalid.
Use case ends.
- 2b1. TAHub shows an error message stating that the Student Index is invalid.
- 2c. Student Name not Found in Student List.
- 2c1. TAHub shows an error message stating that the Student does not exist.
Use case ends.
- 2c1. TAHub shows an error message stating that the Student does not exist.
- 2d. Student is already in consultation
- 2d1. TAHub shows an error message stating that the Student is already in the consultation.
Use case ends.
- 2d1. TAHub shows an error message stating that the Student is already in the consultation.
Use case: UC11 - Remove Student from Consultation
Precondition: The Consultation exists in TAHub.
MSS:
- User requests to remove a specific student from the consultation by providing the necessary details (Consultation Index, Student Name)
- TAHub validates the inputs.
- TAHub removes the student from the consultation.
- TAHub displays the updated consultation list.
Use case ends.
Extensions:
- 2a. Invalid Consultation Index.
- 2a1. TAHub shows an error message stating that the Consultation Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Consultation Index is invalid.
- 2b. Student Name not Found in Student List.
- 2b1. TAHub shows an error message stating that the Student does not exist.
Use case ends.
- 2b1. TAHub shows an error message stating that the Student does not exist.
- 2c. Student is not in consultation.
- 2c1. TAHub shows an error message stating that the Student is not in the consultation.
Use case ends.
- 2c1. TAHub shows an error message stating that the Student is not in the consultation.
Use case: UC12 - Delete Consultation
Precondition: The Consultation exists in TAHub.
MSS:
- User requests to delete a specific consultation by providing the necessary details (Consultation Index).
- TAHub verifies the Consultation Index.
- TAHub deletes the consultation at the index in the Consultation List.
Use case ends.
Extensions:
- 2a. Invalid Consultation Index.
- 2a1. TAHub shows an error message stating that the Consultation Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Consultation Index is invalid.
Use case: UC13 - Export Consultation Data
MSS:
- User requests to export consultation data with desired filename.
- TAHub validates filename.
- TAHub creates CSV file with consultation data.
- TAHub copies file to home directory.
- Success message shows number of consultations exported.
Use case ends.
Extensions:
- [Same extensions as UC6]
Use case: UC14 - Import Consultation Data
MSS:
- User requests to import consultation data with CSV filename.
- TAHub validates file exists and is readable.
- TAHub reads CSV header and validates format.
- TAHub processes each row:
- Validates date and time format.
- Checks student existence in TAHub.
- Creates consultation entries.
- TAHub shows number of consultations imported and any errors.
Use case ends.
Extensions:
- 2a. File not found.
- 2a1. TAHub shows error message about missing file.
Use case ends.
- 2a1. TAHub shows error message about missing file.
- 3a. Invalid header format.
- 3a1. TAHub shows error message about expected format
Use case ends.
- 3a1. TAHub shows error message about expected format
- 4a. Invalid data in rows
- 4a1. TAHub logs invalid entries to error.csv.
- 4a2. TAHub continues processing remaining rows.
- 4a3. Error types include:
- Invalid date/time format.
- Student not found in TAHub.
- Duplicate consultation.
- 4a4. Success message includes count of errors.
Use case ends.
Use case: UC15 - Refresh Consultation List
Guarantees:
- Overall Consultation List will be displayed.
MSS:
- User requests to refresh consultation list.
- TAHub refreshes and displays the consultation list.
Use case ends.
Use case: UC16 - Create a Lesson
MSS:
- User requests to add a lesson by providing the necessary details (date, time).
- TAHub validates the inputs.
- TAHub adds the lesson with the provided details.
- TAHub displays the updated lesson list.
Use case ends.
Extensions:
- 2a. One or more input parameters are missing or invalid.
- 2a1. TAHub shows an error message indicating the missing or invalid fields.
Use case ends
- 2a1. TAHub shows an error message indicating the missing or invalid fields.
- 2b. Duplicate Lesson Exists (Lesson date & time matches an existing lesson)
- 2b1. TAHub shows a duplicate error message.
Use case ends.
- 2b1. TAHub shows a duplicate error message.
Use case: UC17 - Add Student to Lesson
Precondition: The Lesson exists in TAHub.
MSS:
- User requests to add a specific student to the lesson by providing the necessary details (Lesson Index, Student Index, Student Name)
- TAHub validates the inputs.
- TAHub adds the student to the lesson.
- TAHub displays the updated lesson list.
Use case ends.
Extensions:
- 2a. Invalid Lesson Index.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
- 2b. Invalid Student Index.
- 2b1. TAHub shows an error message stating that the Student Index is invalid.
Use case ends.
- 2b1. TAHub shows an error message stating that the Student Index is invalid.
- 2c. Student Name not Found in Student List.
- 2c1. TAHub shows an error message stating that the Student does not exist.
Use case ends.
- 2c1. TAHub shows an error message stating that the Student does not exist.
- 2d. Student is already in lesson.
- 2d1. TAHub shows an error message stating that the Student is already in the lesson.
Use case ends.
- 2d1. TAHub shows an error message stating that the Student is already in the lesson.
Use case: UC18 - Remove Student from Lesson
Precondition: The Lesson exists in TAHub.
MSS:
- User requests to remove a specific student from the lesson by providing the necessary details (Lesson Index, Student Name)
- TAHub validates the inputs.
- TAHub removes the student from the lesson.
- TAHub displays the updated lesson list.
Use case ends.
Extensions:
- 2a. Invalid Lesson Index.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
- 2b. Student Name not Found in Student List.
- 2b1. TAHub shows an error message stating that the Student does not exist.
Use case ends.
- 2b1. TAHub shows an error message stating that the Student does not exist.
- 2c. Student is not in lesson
- 2c1. TAHub shows an error message stating that the Student is not in the lesson.
Use case ends.
- 2c1. TAHub shows an error message stating that the Student is not in the lesson.
Use case: UC19 - Delete Lesson
Precondition: The Lesson exists in TAHub.
MSS:
- User requests to delete a specific lesson by providing the necessary details (Lesson Index).
- TAHub verifies the Lesson Index.
- TAHub deletes the lesson at the index in the Lesson List.
Use case ends.
Extensions:
- 2a. Invalid Lesson Index
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
Use case: UC20 - Mark Student Attendance in Lesson
Precondition: The Student & Lesson exists in TAHub. Student is already in the Lesson.
MSS:
- User requests to mark a specific student’s attendance in the lesson by providing the necessary details (Lesson Index, Student Name, Attendance)
- TAHub validates the inputs.
- TAHub marks the student’s attendance in the lesson.
- TAHub displays the updated student attendance.
Use case ends.
Extensions:
- 2a. Invalid Lesson Index.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
- 2b. Student is not in lesson.
- 2b1. TAHub shows an error message stating that the Student is not in the lesson.
Use case ends.
- 2b1. TAHub shows an error message stating that the Student is not in the lesson.
- 2c. Invalid Attendance.
- 2c1. TAHub shows an error message stating that the Attendance is invalid.
Use case ends.
- 2c1. TAHub shows an error message stating that the Attendance is invalid.
Use case: UC21 - Mark Student Participation in Lesson
Precondition: The Student & Lesson exists in TAHub. Student is already in the Lesson.
MSS:
- User requests to mark a specific student’s participation in the lesson by providing the necessary details (Lesson Index, Student Name, Participation)
- TAHub validates the inputs.
- TAHub marks the student’s participation in the lesson.
- TAHub displays the updated student attendance & participation.
Use case ends.
Extensions:
- 2a. Invalid Lesson Index.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
Use case ends.
- 2a1. TAHub shows an error message stating that the Lesson Index is invalid.
- 2b. Student is not in lesson.
- 2b1. TAHub shows an error message stating that the Student is not in the lesson.
Use case ends.
- 2b1. TAHub shows an error message stating that the Student is not in the lesson.
- 2c. Invalid Participation.
- 2c1. TAHub shows an error message stating that the Participation is invalid.
Use case ends.
- 2c1. TAHub shows an error message stating that the Participation is invalid.
- 2d. Participation Score is Positive & Valid.
- 2d1. TAHub marks the student’s attendance as Present.
Use case resumes from step 3.
- 2d1. TAHub marks the student’s attendance as Present.
Use case: UC22 - Refresh Lesson List
Guarantees:
- Overall Lesson List will be displayed.
MSS:
- User requests to refresh lesson list.
- TAHub refreshes and displays the lesson list.
Use case ends.
Non-Functional Requirements
- Should work on any mainstream OS as long as it has Java
17
or above installed. - Should be able to hold up to 1000 students without a noticeable sluggishness in performance for typical usage.
- A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
- The system should be able to scale to accommodate a growing number of users (teachers, TAs, students) without requiring significant reengineering.
- The platform should have a clean, intuitive user interface that allows new users to complete basic tasks (like searching for a student) with minimal training.
- Any search query should return results within 1 second for up to 10,000 student records.
- Product should be for a single user.
- The data should be stored locally and should be in a human editable text file.
- The software should work without requiring an installer.
Planned Enhancements
Make command names case-insensitive
Description
Make command names case-insensitive, i.e. Find
is safe to substitute for
find
in the find
command.
Rationale
Users may make small mistakes in capitalisation when typing quickly. It can save time to ensure minor mistakes such as these in the command keyword (not the arguments) will not prevent the command from working.
Allow students with same name to be added
Description
Allow students with the same name to be added (still case-sensitive).
Instead, disallow students with the same phone number or email address to be added.
Commands that currently use student names as arguments should instead use their index
in the student list.
Rationale
It is arguably rarer for students to share a phone number or email address (university email?) with another student than it is to share a name.
Allow more commands to use student index instead of full name
Description
Currently, some commands such as marka
and markp
require the user to type
out the full name of the student.
Instead, change it so that they use the student’s index in the student list, similar to commands
like addtolesson
.
Rationale
Under normal conditions, it is impossible for a consultation or lesson to have a student
that is not in the student list. Thus, it is safe to specify students by their index
in the student list.
Additionally, doing so is faster to type.
Allow certain special characters to be used in names
Description
Allow more special characters such as /
and -
to be used in student names.
Rationale
It is possible for students’ legal names to contain -
(e.g. Mary-Ann) or /
(e.g. S/O).
Relaxing current restrictions to allow such characters will allow such names to be input.
Add clearer error message for integers/indexes
Description
Currently, when an invalid or sufficiently large number is given as an index, the error message says:
Index is not an unsigned non-zero integer.
This should be changed to specify the requirement that indexes should be between
1 and Integer.MAX_VALUE
.
Rationale
The current error message can be confusing for non-technical users who do not know what
unsigned means, and misleading when it also shows for large inputs that exceed Java’s
integer limit, such as 104890385925902379
.
Clearer error messages can help to mitigate such confusion.
Make `find c/` throw an error
Description
Currently, find c/
does not throw an error. Instead, it runs successfully but always
returns 0 students.
This should be changed so an appropriate error message is shown (courses cannot be empty).
Rationale
A user might expect find c/
to find students who are taking no courses.
However, this is not the case, and will result in confusion.
Hence, this command should not execute successfully.
Make participation not accept + before the number
Description
Currently, the participation argument in the markp
command accepts the use of +
before it,
i.e. +3
is treated as 3
.
This should be treated as an invalid format.
Rationale
Though not strictly wrong, the index parser currently already checks for +
and treats it as invalid.
For consistency, this should also apply to participation.
Make year 0000 an illegal value for the date
Description
Currently, year 0000 is accepted as a valid year. This should be changed to no longer be valid.
Rationale
According to Wikipedia, a year 0 does not exist in the Anno Domini calendar year. Therefore, it should not be allowed.
Standardise error messages involving index
Description
For some commands, entering specific indexes (like 0) will show an error message stating that the index is invalid (not an unsigned nonzero integer), while other times it will show the default error message for incorrect format.
Rationale
These error messages should be standardised to avoid confusion. Any errors when parsing the index specifically ideally should specify that the index specifically is invalid to help the user correct it.
Glossary
- Attendance: Student’s Presence/Absence for a Lesson
- Consultation: A scheduled meeting between TA and students for academic discussions
- Lesson: An Official Tutorial/Lab coordinated by TA
- Mainstream OS: Windows, Linux, Unix, MacOS
- Participation: Student’s Score for performance in a Lesson
- Private contact detail: A contact detail that is not meant to be shared with others
- Student*: A Person with Name, Contact, Email & Courses
- Student Record: A collection of data fields that stores information about a student, including their name, contact information, course enrollment
- TA: Teaching Assistant
Appendix: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
Student Test Cases
Adding a student
-
Test case:
add n/TestOne p/11111111 e/test1@example.com c/CS2103T
Expected: StudentTestOne
is added to the list. Details of the added student is shown. -
Test case:
add n/TestOne p/11111111
Expected: No student is added. Error details shown. -
Test case:
add n/TestOne e/test1@example.com c/CS2103T
Expected: No student is added. Error details shown. -
Test case:
add n/Test1 p/11111111 e/test1@example.com c/CS2103T
Expected: No student is added. Error details shown.
Finding a student (by course)
-
Prerequisites: List all students using the
liststudents
command. Multiple students in the list. -
Test case:
find c/CS2103T
(Assuming Students with courseCS2103T
Exist)
Expected: Displays students details with courseCS2103T
. -
Test case:
find c/CS2103T
(Assuming Students with courseCS2103T
does not Exist)
Expected: No Students Found. Displays 0 students. -
Test case:
find c/1234
Expected: No Students Found. Error details shown.
Finding a student (by name)
-
Prerequisites: List all students using the
liststudents
command. Multiple students in the list. -
Test case:
find n/TestOne
(Assuming Student with nameTestOne
Exists)
Expected: Displays students details with nameTestOne
. -
Test case:
find n/TestOne
(Assuming Students with nameTestOne
does not Exist)
Expected: No Students Found. Displays 0 students. -
Test case:
find n/Test1
Expected: No Students Found. Error details shown.
Editing a student
-
Prerequisites: List all students using the
liststudents
command. Multiple students in the list. -
Test case:
edit 1 n/TestOne p/11111111
Expected: 1st student is edited. Details of the edited student is shown. -
Test case:
edit 2 e/test1@example.com c/CS2103T
Expected: 2nd student is edited. Details of the edited student is shown. -
Test case:
edit 2 n/Test 2
Expected: No student is edited. Error details shown. -
Other incorrect edit commands to try:
edit
,edit x
,...
(where x is larger than the list size)
Expected: No student is edited. Error details shown.
Deleting a student
-
Prerequisites: List all students using the
liststudents
command. Multiple students in the list. -
Test case:
delete 1
Expected: 1st student is deleted from the list. Details of the deleted student shown in the status message. -
Test case:
delete 0
Expected: No student is deleted. Error details shown in the status message. -
Other incorrect delete commands to try:
delete
,delete x
,...
(where x is larger than the list size)
Expected: No student is deleted. Error details shown in the status message.
Lesson Test Cases
Adding a lesson: addlesson
-
Test case:
addlesson d/2024-10-20 t/14:00
Expected: Lesson with date 2024-10-20 and time 14:00 is added to the list. Details of the added lesson are shown. -
Test case:
addlesson d/2024-10-20
Expected: No lesson is added. Error details shown. -
Test case:
addlesson t/14:00
Expected: No lesson is added. Error details shown. -
Test case:
addlesson d/2024-10-20 t/14:00
(when a lesson with the same date and time already exists)
Expected: No lesson is added. Error message about duplicate lesson shown.
Adding a student to a lesson: addtolesson
-
Prerequisites: At least one lesson and one student exist in the list.
-
Test case:
addtolesson 1 n/TestOne
Expected: Student TestOne is added to the first lesson. Confirmation message is shown. -
Test case:
addtolesson 1 i/2
(assuming the student at index 2 exists)
Expected: The student at index 2 is added to the first lesson. Confirmation message is shown. -
Test case:
addtolesson 1 n/NonExistentStudent
Expected: No student is added. Error message about student not found shown. -
Test case:
addtolesson 2 n/TestOne
(where lesson at index 2 doesn’t exist)
Expected: No student is added. Error details shown. -
Test case:
addtolesson 1 n/TestOne i/2
Expected: Both TestOne and the student at index 2 are added to the first lesson. Confirmation message is shown.
Removing a student from a lesson: removefromlesson
-
Prerequisites: At least one lesson with one or more students exists in the list.
-
Test case:
removefromlesson 1 n/TestOne
Expected: Student TestOne is removed from the first lesson. Confirmation message is shown. -
Test case:
removefromlesson 1 n/NonExistentStudent
Expected: No student is removed. Error message about student not found shown. -
Test case:
removefromlesson 2 n/TestOne
(where lesson at index 2 doesn’t exist)
Expected: No student is removed. Error details shown.
Marking attendance in a lesson: marka
-
Test case:
marka 1 n/TestOne a/1
Expected: StudentTestOne
is marked as attended for the first lesson. Confirmation message is shown. -
Test case:
marka 1 n/TestOne a/0
Expected: StudentTestOne
is marked as absent for the first lesson. Confirmation message is shown. -
Test case:
marka 1 n/John Doe n/Jane Doe a/1
Expected: StudentsJohn Doe
andJane Doe
are both marked as attended for the first lesson. Confirmation message is shown. -
Test case:
marka 1 n/John Doe n/Jane Doe a/0
Expected: StudentsJohn Doe
andJane Doe
are both marked as absent for the first lesson. Confirmation message is shown. -
Test case:
marka 1 n/NonExistentStudent a/1
Expected: No attendance is marked. Error message about student not found is shown. -
Test case:
marka 2 n/TestOne a/1
(where lesson at index 2 doesn’t exist)
Expected: No attendance is marked. Error message about lesson not found is shown.
Marking participation in a lesson: markp
-
Test case:
markp 1 n/TestOne pt/10
Expected: StudentTestOne
is marked with a participation score of 10 for the first lesson, and attendance is set to true. Confirmation message is shown. -
Test case:
markp 1 n/TestOne pt/0
Expected: StudentTestOne
is marked with a participation score of 0 for the first lesson. Attendance remains unchanged. Confirmation message is shown. -
Test case:
markp 1 n/John Doe n/Jane Doe pt/15
Expected: StudentsJohn Doe
andJane Doe
are both marked with a participation score of 15 for the first lesson, and their attendance is set to true. Confirmation message is shown. -
Test case:
markp 1 n/NonExistentStudent pt/10
Expected: No participation is marked. Error message about student not found is shown. -
Test case:
markp 2 n/TestOne pt/10
(where lesson at index 2 doesn’t exist)
Expected: No participation is marked. Error message about lesson not found is shown. -
Test case:
markp 1 n/TestOne pt/101
Expected: No participation is marked. Error message about invalid participation score is shown (as the score exceeds the valid range of 0-100). -
Test case:
markp 1 n/TestOne pt/-1
Expected: No participation is marked. Error message about invalid participation score is shown (as the score is below the valid range of 0-100).
Removing a lesson: deletelesson
-
Prerequisites: At least one lesson exists in the list.
-
Test case:
deletelesson 1
Expected: The first lesson is removed from the list. Confirmation message is shown. -
Test case:
deletelesson 0
Expected: No lesson is removed. Error details shown. -
Other incorrect remove commands:
deletelesson
,deletelesson x
(where x is larger than the list size)
Expected: No lesson is removed. Error details shown. -
Test case:
deletelesson 1;2
Expected: Both the 1st and 2nd lessons are deleted from the list. Confirmation message is shown.
Consultation Test Cases
Adding a consultation: addconsult
-
Test case:
addconsult d/2024-10-20 t/14:00
Expected: Consultation on 2024-10-20 at 14:00 is added. Confirmation message is shown. -
Test case:
addconsult d/2024-10-20
Expected: No consultation is added. Error details shown. -
Test case:
addconsult t/14:00
Expected: No consultation is added. Error details shown. -
Test case:
addconsult d/2024-10-20 t/14:00
(when a consultation with the same date and time exists)
Expected: No consultation is added. Error message about duplicate consultation shown.
Listing all consultations: listconsults
- Prerequisites: At least one consultation exists.
- Test case:
listconsults
Expected: Displays a list of all consultations.
Adding students to a consultation: addtoconsult
-
Prerequisites: At least one consultation and one student exist.
-
Test case:
addtoconsult 1 n/TestOne
Expected: Student TestOne is added to the first consultation. Confirmation message is shown. -
Test case:
addtoconsult 1 i/2
(assuming the student at index 2 exists)
Expected: The student at index 2 is added to the first consultation. Confirmation message is shown. -
Test case:
addtoconsult 1 n/NonExistentStudent
Expected: No student is added. Error message about student not found shown. -
Test case:
addtoconsult 2 n/TestOne
(where consultation at index 2 doesn’t exist)
Expected: No student is added. Error details shown. -
Test case:
addtoconsult 1 n/TestOne i/2
Expected: Both TestOne and the student at index 2 are added to the first consultation. Confirmation message is shown.
Removing students from a consultation: removefromconsult
-
Prerequisites: At least one consultation with one or more students exists.
-
Test case:
removefromconsult 1 n/TestOne
Expected: Student TestOne is removed from the first consultation. Confirmation message is shown. -
Test case:
removefromconsult 1 n/NonExistentStudent
Expected: No student is removed. Error message about student not found shown. -
Test case:
removefromconsult 2 n/TestOne
(where consultation at index 2 doesn’t exist)
Expected: No student is removed. Error details shown.
Deleting consultations: deleteconsult
-
Prerequisites: At least one consultation exists.
-
Test case:
deleteconsult 1
Expected: The first consultation is deleted. Confirmation message is shown. -
Test case:
deleteconsult 0
Expected: No consultation is deleted. Error details shown. -
Other incorrect delete commands:
deleteconsult
,deleteconsult x
(where x is larger than the list size)
Expected: No consultation is deleted. Error details shown. -
Test case:
deleteconsult 1;2
Expected: Both the 1st and 2nd consultations are deleted from the list. Confirmation message is shown.
Saving data
-
Dealing with missing/corrupted data files
- If you encounter an unexpected empty TAHub (no students, consults or lessons) upon startup or your data is replaced by sample data, your data file may be corrupted.
- If you wish to try and salvage your data, do not perform any command yet. This will overwrite your data.
- Copy your data file to make a safe backup first, and rename it something other than
addressbook
. You can open this file to view your data in JSON format. - TAHub will generate a new data file with sample data. In the meantime, if you are experienced with JSON, you can attempt to recover your data file by fixing issues in the file, usually syntax/formatting.
Exporting data
Exporting student data
-
Prerequisites: List all students using the
liststudents
command. Multiple students should be in the list. -
Test case:
export students
Expected: CSV file created in data directory and home directory. Success message shows number of students exported. -
Test case:
export test.file
Expected: Error message about invalid filename characters. -
Test case:
export students
(when students.csv already exists)
Expected: Error message suggesting force flag usage. -
Test case:
export -f students
Expected: Existing file overwritten. Success message shows number of students exported.
Exporting consultation data
-
Prerequisites: List all consultations using the
listconsults
command. Multiple consultations should be in the list. -
Test case:
exportconsult sessions
Expected: CSV file created with consultation data. Success message shows number of consultations exported. -
Test case:
exportconsult sessions
(when file exists)
Expected: Error message suggesting force flag usage. -
Other incorrect export commands to try:
exportconsult
,exportconsult /test
,exportconsult test.csv
Expected: Error messages about invalid format/filename.
Importing data
Importing student data
-
Prerequisites: Prepare a valid CSV file with header “Name,Phone,Email,Courses”
-
Test case:
import students.csv
(with valid data)
Expected: Students imported successfully. Success message shows number of students imported. -
Test case:
import nonexistent.csv
Expected: Error message about file not found. -
Test case: Import file with invalid rows (wrong format, duplicate students)
Expected: Some students imported. Error.csv created with invalid entries. Success message shows counts of successes and failures.
Importing consultation data
-
Prerequisites: Prepare a valid CSV file with header “Date,Time,Students”
-
Test case:
importconsult sessions.csv
(with valid data)
Expected: Consultations imported successfully. Success message shows number of consultations imported. -
Test case: Import file with invalid dates or times
Expected: Invalid entries logged to error.csv. Success message shows counts. -
Test case: Import file with nonexistent students
Expected: Entries with invalid students logged to error.csv. Success message shows counts. -
Other incorrect import commands to try:
importconsult
,importconsult /test.csv
,importconsult ../test.csv
Expected: Error messages about invalid format or file location.